P.S You may also interested in this Spring Boot JDBC Examples. Query for Single Row. In Spring, we can use jdbcTemplate.queryForObject to query a single row record from database, and convert the row into an object via row mapper. Spring 4 JdbcTemplate Annotation Example. By Yashwant Chavan, Views 558927, Last updated on 15-Dec-2016. In this tutorial, we will learn how to connect to the database and execute CRUD SQL queries using Spring 4 JdbcTemplate. In this post we will see how to select records using queryForObject, queryForList, BeanPropertyRowMapper in Spring JdbcTemplate.Here we will create annotation based example.
In this Spring CRUD Example, we will build a Simple Spring Application and perform CRUD operations using Spring JdbcTemplate. We will create a simple Employee management application which has abilities to create a new employee, update the existing employee, get a particular employee/ all employee and finally delete the existing employee.
Creating table
Create EMPLOYEETable, simply Copy and Paste the following SQL query in the query editor to get the table created.
Folder Structure:
- Create a simple Maven Project “SpringJDBC”by selecting maven-archetype-quickstart and create a package for our source files “com.javainterviewpoint” under src/main/java
- Now add the following dependency in the POM.xml
- Create the Java classes Employee.java,EmployeeDAOImpl.java andSpringJDBCExample.java under com.javainterviewpointfolder.
Other interesting articles which you may like …
Spring CRUD Example
Employee.java
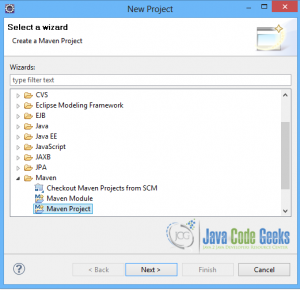
Our Employee class is a simple POJO class consisting getters and setters of Employee properties id, name, age, dept.
SpringConfig.xml
In our configuration file, we have defined the three beans
- DriverManagerDataSource – DriverManagerDataSource contains database related configurations such as driver class name, connection URL, username and password.
- JdbcTemplate – We will be referencing the dataSource id (DriverManagerDataSource ) to the property dataSource of the JdbcTemplate class.
- EmployeeDAOImpl – We will be referencing the jdbcTemplate id to the property jdbcTemplate of the EmployeeDAOImpl class.

EmployeeDAO.java
EmployeeDAOImpl.java
EmployeeDAOImpl class implements the interface EmployeeDAO and overrides all the unimplemented methods. We have the below methods in our EmployeeDAOImpl class
- setJdbcTemplate() – Through Spring setter injection we will be injecting the jdbcTemplate from the Spring configuration file.
- getAllEmployee() – In order to fetch all the records from the database we just need to pass the SQL and the instance of the ResultSetExtractor to the query() method of jdbcTemplate. ResultSetExtractor interface accepts the ResultSet and returns a Java List. We need to override the extractData() method and map each ResultSet to an Employee object add to a list.
- getEmployeeById() – In order to fetch a particular record we just need to pass the SQL and the instance of the RowMapper to the queryForObject() method of jdbcTemplate. RowMapper interface internally iterates the ResultSet and adds it to the Collection (Map). Hence there is no need for us to iterate the ResultSet as we do in the ResultSetExtractor.
- updateEmployee() – We will be updating the corresponding employee by calling the update() method of the jdbcTemplate passing the SQL and the parameters.
- deleteEmployee() – In order to delete an employee, we need to call the update() method of the jdbcTemplate passing the SQL and the id.
SpringJDBC.java

- ClassPathXmlApplicationContext class reads our Configuration File(SpringConfig.xml)
- We will get our EmployeeDAOImpl Class instance by calling the getBean() method over the context.
- Call the saveEmployee(), getEmployeeById(), getAllEmployees(),updateEmployee() and deleteEmployee() methods over the EmployeeDAOImpl instance which we got above.
Output:
Select For Update Mysql
Download Source Code
In this example you will learn how to select records from the database using JdbcTemplate.queryForList()
method. This method returns a List
object which stores information selected from the table in a HashMap
object. The key
of the map is the table’s field names while the value
of the map contains the corresponding table’s field value.
Oracle Sql Select For Update
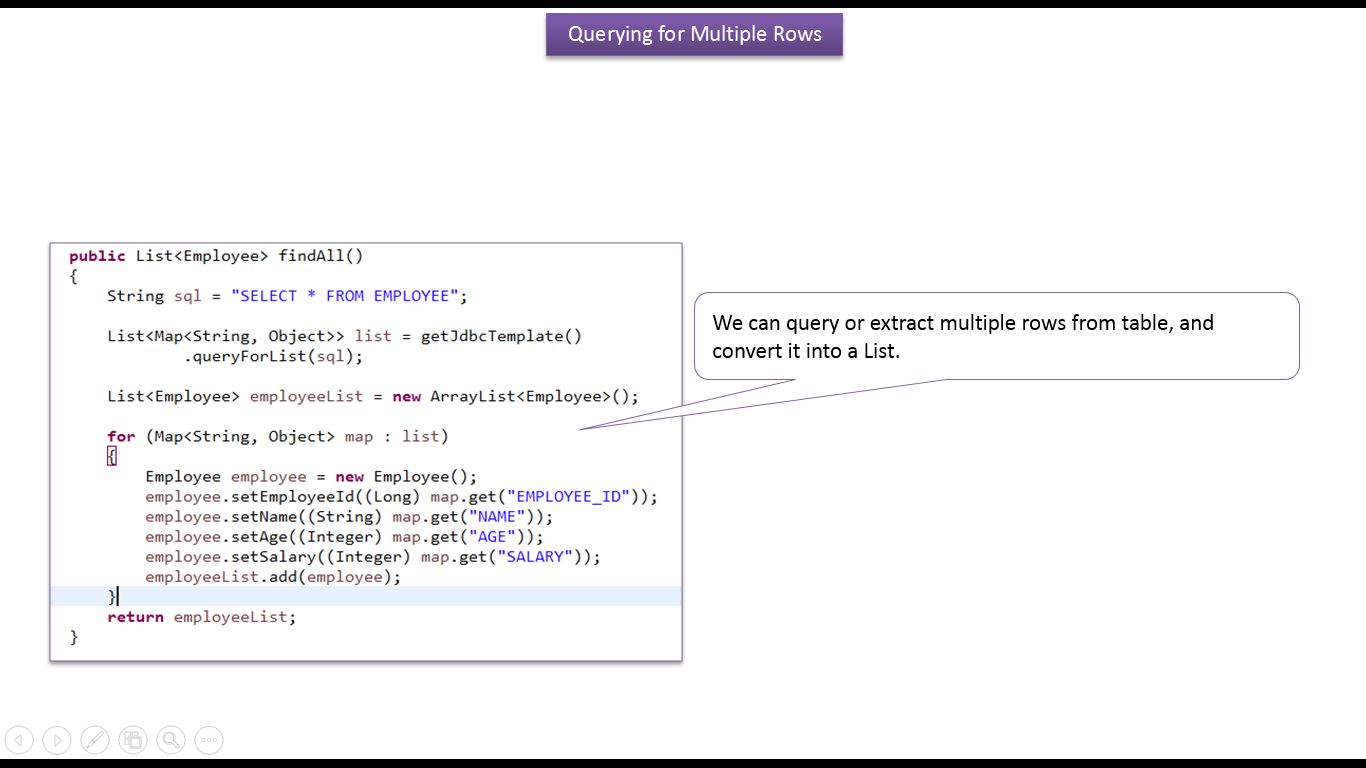
Spring Jdbctemplate Select For Update Example
- How do I convert java.util.TimeZone to java.time.ZoneId? - April 25, 2020
- How do I get a list of all TimeZones Ids using Java 8? - April 25, 2020
- How do I get HTTP headers using HttpClient HEAD request? - April 22, 2020